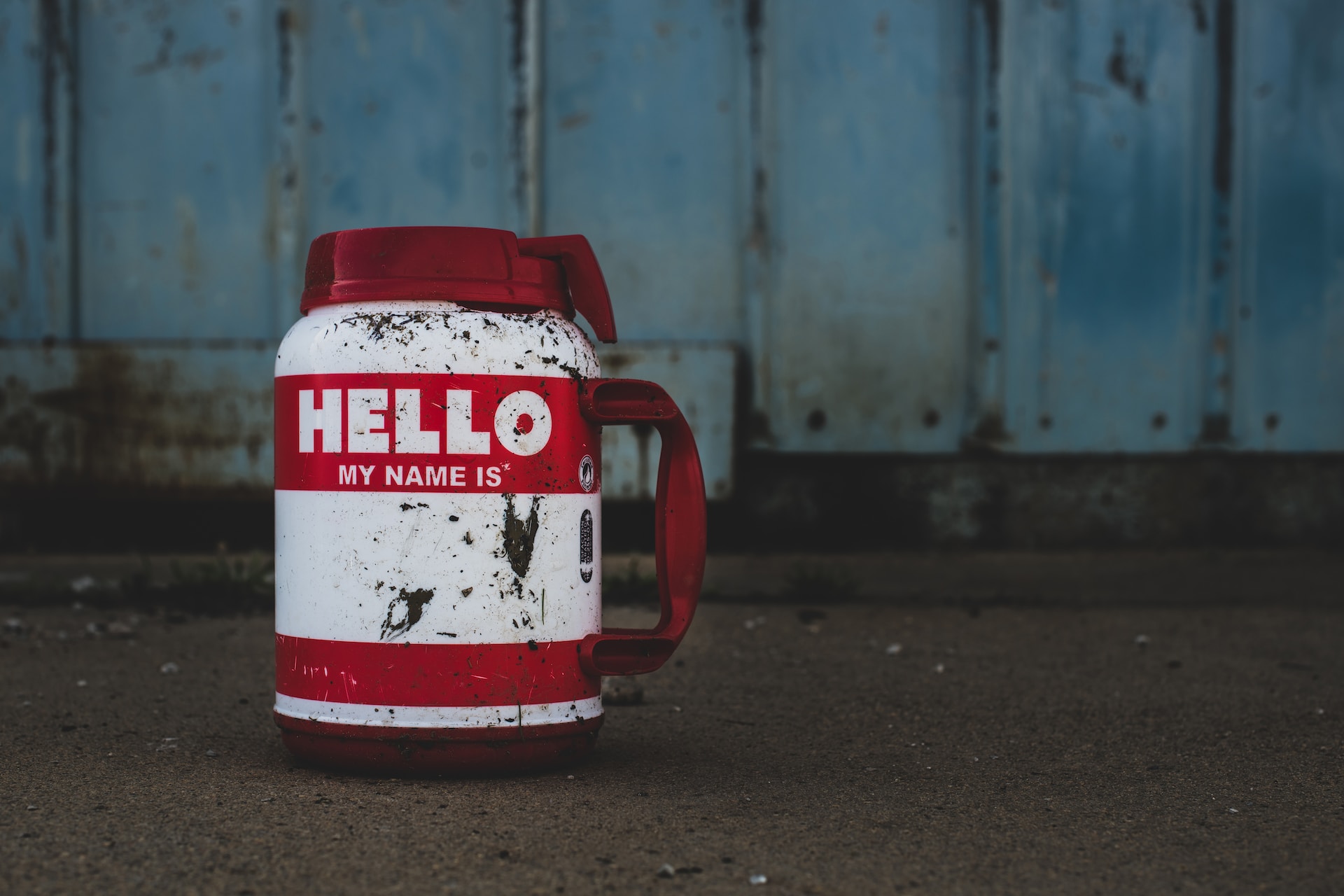
SuiteScript Function Naming
The examples provided in this article pertain to SuiteScript 1.0. With the introduction of SuiteScript 2.0 and 2.1, a more modular pattern is available that makes the idea of namespaces less necessary.
Over time, everyone builds their own style of programming. A lot of that style is defined by your past experience, where and how you learn a particular concept, the context in which the code is being written and your goals when writing that code. There are many styles of programming and I would not consider any of them to be right or wrong because there are pros and cons to each way of getting the job done.
When writing scripts for NetSuite there are a few things to keep in mind. What do I name my function? When is the script going to execute? What is the script going to do? Let’s talk about naming our functions.
A function by any other name
The names that we choose for our functions in SuiteScript are important because there are essentially only two scopes for naming variables and functions, local and global scope. Any functions that we define that are in the global scope are available everywhere. By that same token, any functions defined in the global scope by any third party bundles that you’ve installed also exist everywhere. And in javascript, the code that defines the function last, wins.
Probably not a huge deal with third party vendors but as your script library grows, wouldn’t it be nice to be able to reuse our own code across multiple scenarios? When we define a script record in NetSuite, we can list the Libraries, or other script files, that will be loaded before the current script is executed. If we have two functions defined with the same name in the global scope, the last function defined will override any previous definitions. Consider the following BeforeSubmit function definition:
function beforeSubmit(type) {
// Validate customer data before saving record
}
With a function name this generic, it’s more likely that we have other functions defined in other SuiteScript files with the same name. This means that we probably can’t use this file as a library for another script. There could be a naming conflict so let’s put our function into a ‘namespace’ so that our beforeSubmit()
function is locally scoped rather than globally.
This very simple namespace pattern in Javascript will help ensure that our function does not exist in the global scope and isn’t likely to clash with any other files we might want to include as libraries:
var DT = DT || {};
DT.customer = DT.customer || {};
DT.customer.validate = {
beforeSubmit : function(type) {
// Validate customer data before saving record
}
};
Essentially, the beginning of this script either builds a new namespace or adds this functionality to a namespace of the same name if one has already been defined.
The first line starts our namespace by asking if there is already a variable named DT
in the global scope. If there is, give us that variable. If not, create an empty object.
The second line asks if there is a customer
property that already exists on the DT
variable. If there is, give us that variable. If not, make the customer
property an empty object.
The rest of this script defines a validate
property on customer and sets it’s value to an object that has a beforeSubmit
property which happens to be a function.
In this script, the beforeSubmit()
function is not defined in the global scope so it can only clash with another function named DT.customer.validate.beforeSubmit()
. That’s much less likely to happen.
Following this pattern in each SuiteScript file helps to build a common structure for all of our files. We can then include these files as libraries for other scripts and you’ll know that any one beforeSubmit()
function isn’t going to override a function with the same name in another namespace.
My next article will demonstrate using this namespace technique to build a reusable script to create sales orders.